Type conversions for C/C++
Type conversions
เป็นการแปลงข้อมูลประเภทหนึ่งไปเป็นอีกประเภทหนึ่งแบ่งออกเป็น 2 แบบใหญ่ๆดังนี้
Implicit type conversion
การแปลงข้อมูลอัตโนมัติจะเกิดขึ้นจากการกำหนดค่าตัวแปร หรือ การคัดลอกตัวแปรหนึ่งไปยังอีกตัวแปรหนึ่ง โดยตัว compiler จะแปลงตัวแปรนั้นให้เป็นประเภทตัวแปรที่สามารถทำงานร่วมกันได้อัตโนมัติ ตัวอย่างเช่น เมื่อกำหนดให้ x เป็นตัวแปรประเภท int มีค่าเท่ากับ 1 และ y เป็น char มีค่าเป็น ‘A’ แต่เมื่อนำค่าตัวแปร x และ y บวกกันโดยผลลัพท์ที่ได้ไปเก็บไว้ใน x ซึ่งเป็น ตัวแปรประเภท int โปรแกรมจะแปลงให้ y เป็นข้อมูลประเภท char ให้เป็น int อัตโนมัติ ดังตัวอย่าง โปรแกรมด้านล่าง
// Ex.1 print Implicit type conversion
#include <iostream>
using namespace std;
int main(){
int x = 1;
char y = 'A';
cout<<"x = "<< x <<", y = " << y<< endl;
// y implicitly converted to int. ASCII
// value of 'a' is 97
x = x+y ;
cout<<" x = "<<x<< endl;
// x is implicitly converted to float
float z = x+1.1;
cout <<" z = "<<z<< endl;
// z is implicitly converted to int
int a = z +1;
cout <<" a = "<<a<< endl;
// a is int of datatype
a = x/a;
cout <<" a = "<<a<< endl;
// a is implicitly converted to float
float b =a;
cout <<" b = "<<b<< endl;
// z is implicitly converted to char
char c = z;
cout <<" c = "<<c<< endl;
return 0;
}
Output
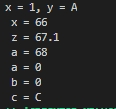
แต่สิ่งที่ต้องระวังสำหรับการเกิดการแปลงอัตโนมัติ คือ
การหารระหว่างตัวแปรประเภทจำนวนเต็ม ถึงแม้จะเกิดจะเกิดค่าทศนิยม แต่ถ้าแปลงเป็น float ตัวเลขชุดนั้นก็จะสูญเสียข้อมูลทศนิยมนั้นไป
การแปลงแบบ Implicit type conversion ทำได้เฉพาะประเภทตัวแปรพื้นฐานเท่ากัน
Explicit type conversion
Explicit type conversion หรือเรียกอีกชื่อว่า Type casting มีสองแบบคือ
แบบ function สามารถเแปลงได้โดยให้ใส่ชื่อตัวแปรที่ต้องการแปลงลงในวงเล็บของประเภทตัวแปร และหน้าวงเล็บเป็นประเภทตัวแปรที่ต้องการแปลง type (variable) ตัวอย่าง int(variable)
แบบ c-likeสามารถเแปลงได้โดยให้ใส่ประเภทตัวแปรที่ต้องการลงในวงเล็บ และหลังวงเล็บปิดให้ใส่ชื่อตัวแปรที่ต้องการแปลง (type)variable ตัวอย่าง (int)variable
// Ex.2 Explicit type conversion
#include <iostream>
using namespace std;
int main(){
double x = 1.1;
char y = 'A';
int b =int(y); // แบบ function
int c = (int)x+1; // แบบ c-like
cout <<" b = "<< b <<endl
<<" c = " << c<< endl;
return 0;
}
แหล่งอ้างอิง:
Last updated
Was this helpful?