Contour
Contour คือ การแสดงเส้นรูปร่างหรือเส้นโค้งที่รวมจุดต่อเนื่องทั้งหมด (ตามแนวเขต) ที่มีสีหรือความเข้มเท่ากัน เป็นเครื่องมือที่มีประโยชน์สำหรับการวิเคราะห์รูปร่าง การรับรู้จดจำและการตรวจจับวัตถุ ภาพที่นำมาใช้ควรเป็นภาพไบนารีหรือภาพสีเทา
Find Contours
$ cv2.findContours(image, cv2.RETR_LIST, cv2.CHAIN_APPROX_SIMPLE)
Parameters:
image => ภาพไบนารีหรือภาพระดับสีเทา
mode => CV_RETR_LIST เป็นโหมดพื้นฐานที่ใช้ในการดึงรูปร่างทั้งหมดของวัตถุ
method => CV_CHAIN_APPROX_SIMPLE เป็นเทคนิคที่ใช้ในการบีบอัดจุดซ้ำซ้อน
ยกตัวอย่างเช่น การวาด contour บนสี่เหลี่ยม จุดที่ต่อเนื่องกันของสี่เหลี่ยมจะถูกบีบอัดให้เหลือเพียง 4 จุดที่จำเป็น
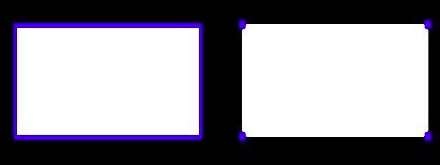
Draw contour
$ cv2.drawContours(image, cnts, contourIdx, color, thickness)
Parameters:
image => ภาพผลลัพธ์ที่ต้องการวาดเส้นกรอบ contour
cnts => รูปทรงอินพุตทั้งหมด แต่ละเส้นจะถูกจัดเก็บเป็นเวกเตอร์จุด
contourIdx => ระบุรูปร่างที่จะวาด หากเป็นลบจะมีการวาดรูปทรงทั้งหมด
color => สีของเส้น contour โดยเขียนในลักษณะ tuple และเป็นลำดับสี BGR
eg: (255, 0, 0) = สีน้ำเงิน
thickness => ความหนาของเส้น ถ้าเป็น -1 จะเป็นกรอบทึบ
Example:
import numpy as np
import cv2
import imutils
image = cv2.imread("/path/your/image.jpg")
image = imutils.resize(image,width = 400)
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
cv2.imshow("Original", image)
cnts = cv2.findContours(gray.copy(), cv2.RETR_LIST, cv2.CHAIN_APPROX_SIMPLE)
cnts = imutils.grab_contours(cnts)
clone = image.copy()
cv2.drawContours(clone, cnts, -1, (0, 255, 0), 2)
cv2.imshow("All Contours", clone)
cv2.waitKey(0)

Centroid
เป็นการหาจุดศูนย์กลางของวัตถุ โดยอาศัยการคำนวณทางคณิตศาสตร์
import numpy as np
import cv2
import imutils
image = cv2.imread("/path/your/image.jpg")
image = imutils.resize(image,width = 400)
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
cv2.imshow("Original", image)
(T, threshInv) = cv2.threshold(gray, 65, 255, cv2.THRESH_BINARY)
cnts = cv2.findContours(threshInv.copy(), cv2.RETR_LIST, cv2.CHAIN_APPROX_SIMPLE)
cnts = imutils.grab_contours(cnts)
clone = image.copy()
for c in cnts:
M = cv2.moments(c)
cX = int(M["m10"] / M["m00"])
cY = int(M["m01"] / M["m00"])
cv2.circle(clone, (cX, cY), 10, (0, 255, 0), -1)
cv2.imshow("All Contours", clone)
cv2.waitKey(0)

Bounding Boxes
import numpy as np
import cv2
import imutils
image = cv2.imread("/path/your/image.jpg")
image = imutils.resize(image,width = 400)
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
cv2.imshow("Original", image)
(T, threshInv) = cv2.threshold(gray, 65, 255, cv2.THRESH_BINARY)
cnts = cv2.findContours(threshInv.copy(), cv2.RETR_LIST, cv2.CHAIN_APPROX_SIMPLE)
cnts = imutils.grab_contours(cnts)
clone = image.copy()
for c in cnts:
(x, y, w, h) = cv2.boundingRect(c)
cv2.rectangle(clone,(x,y),(x+w,y+h),(0,255,0),2)
cv2.imshow("All Contours", clone)
cv2.waitKey(0)

Rotated Bounding Boxes
import numpy as np
import cv2
import imutils
image = cv2.imread("/path/your/image.jpg")
image = imutils.resize(image,width = 400)
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
cv2.imshow("Original", image)
(T, threshInv) = cv2.threshold(gray, 65, 255, cv2.THRESH_BINARY)
cnts = cv2.findContours(threshInv.copy(), cv2.RETR_LIST, cv2.CHAIN_APPROX_SIMPLE)
cnts = imutils.grab_contours(cnts)
clone = image.copy()
for c in cnts:
rect = cv2.minAreaRect(c)
box = cv2.boxPoints(rect)
box = np.int0(box)
cv2.drawContours(clone,[box],0,(0,0,255),2)
cv2.imshow("All Contours", clone)
cv2.waitKey(0)

Minimum Enclosing Circles
import numpy as np
import cv2
import imutils
image = cv2.imread("/path/your/image.jpg")
image = imutils.resize(image,width = 400)
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
cv2.imshow("Original", image)
(T, threshInv) = cv2.threshold(gray, 65, 255, cv2.THRESH_BINARY)
cnts = cv2.findContours(threshInv.copy(), cv2.RETR_LIST, cv2.CHAIN_APPROX_SIMPLE)
cnts = imutils.grab_contours(cnts)
clone = image.copy()
for c in cnts:
(x,y),radius = cv2.minEnclosingCircle(c)
center = (int(x),int(y))
radius = int(radius)
cv2.circle(clone,center,radius,(0,255,0),2)
cv2.imshow("All Contours", clone)
cv2.waitKey(0)

Last updated
Was this helpful?